Accelerating AI Translation 7 Python Optimization Techniques for High-Load Services
Accelerating AI Translation 7 Python Optimization Techniques for High-Load Services - Leveraging PyTorch's 16-bit Precision for Faster Inference
Utilizing PyTorch's 16-bit precision, also known as FP16, can greatly improve the performance of AI translation services, especially when dealing with high volumes of requests. This approach, called mixed precision, combines 16-bit and 32-bit calculations to reduce memory usage and boost computation speed. This can be particularly impactful in AI translation due to the memory intensive nature of large language models and the need for quick responses in applications like machine translation and OCR. Modern GPUs, with Tensor Cores specifically, can see notable speed gains when working with 16-bit precision, making it a viable approach for pushing the boundaries of what is achievable within limited computing resources.
While beneficial, this optimization path isn't without its nuances. Effective memory management is critical when using 16-bit precision, particularly in regards to how key-value caches are handled. As the batch size and sequence length increase, the memory consumption grows, potentially creating a bottleneck if not addressed properly. Fortunately, ongoing advancements within PyTorch aim to streamline mixed precision operations, promising further improvements in the speed and efficiency of generative AI models like those utilized for real-time AI translation. This push for optimization can potentially unlock new possibilities for the widespread implementation of these technologies in services like fast translation and AI-powered OCR tools.
Utilizing 16-bit precision (FP16) instead of the standard 32-bit (FP32) in PyTorch can potentially double the speed of inference, particularly on hardware designed for it. This translates to faster processing of AI-powered translation tasks, which is critical for services needing high throughput. This benefit is amplified when dealing with large datasets common in AI translation, especially when the goal is cheap and fast translations.
PyTorch's mixed precision approach allows us to combine 16-bit and 32-bit representations, leading to reduced memory usage without necessarily compromising accuracy. This is quite useful for handling the extensive data associated with machine translation and OCR systems, especially when computational resources are limited. However, careful management of the key-value cache is crucial as its size increases with both batch size and sequence length, which can become a bottleneck.
Interestingly, some researchers are exploring lower-precision weight formats (like 4-bit). Dequantization of these weights can occur within the GPU cache during matrix multiplications as a 16-bit operation. This idea could be a promising path towards optimized memory and computational efficiency, but it’s still an area of active investigation.
PyTorch provides a convenient way to leverage this speed boost as it can automatically execute operations using FP16 when possible. This simplifies integration, making it a more accessible approach for developers. But, using reduced precision introduces new considerations, primarily the risk of gradient underflow during training. Employing a dynamically scaled loss function is essential to counter this issue.
While the idea is attractive, we must acknowledge a potential trade-off: sometimes, sacrificing precision can make the model more difficult to understand and analyze. This issue is relevant to OCR tasks and other scenarios where interpretation is important. Furthermore, implementing 16-bit precision, while offering speed enhancements, might not always be a straightforward fix. Thorough testing is necessary to ensure that the chosen approach doesn't compromise the quality of AI translations.
However, the current trend in hardware favors the adoption of 16-bit precision as GPUs become increasingly optimized for it. Early tests show that accuracy sacrifices are often minimal (often below 1% accuracy drop) for many NLP tasks, making this approach more enticing for various applications. This shift, coupled with the potential cost savings from lower memory requirements and the possibility of faster translation of low-resource languages, shows promise. We are constantly witnessing new PyTorch features aimed at improving generative AI performance, especially with native PyTorch implementations, and these features will likely play a big role in further refining the efficacy of 16-bit precision in future AI translation systems.
Accelerating AI Translation 7 Python Optimization Techniques for High-Load Services - Implementing Asynchronous Processing with asyncio
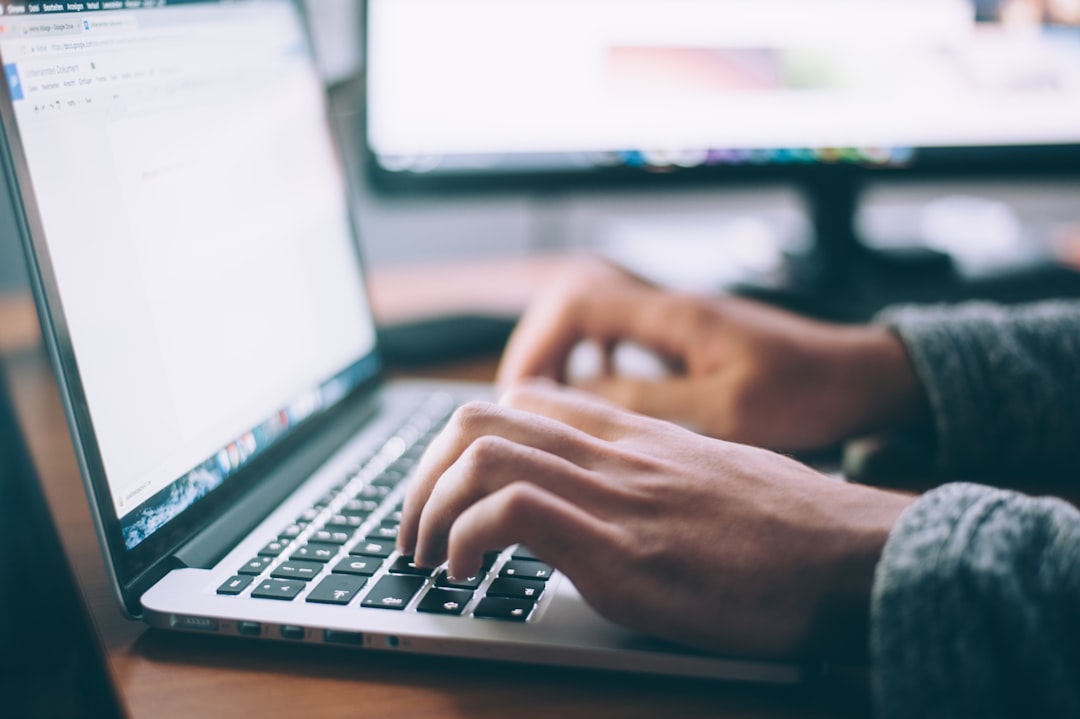
Asynchronous processing, made possible by Python's `asyncio` library, emerges as a valuable technique for optimizing AI translation services, particularly those dealing with high volumes of requests. `asyncio` achieves this by enabling non-blocking operations, allowing multiple translation tasks to run concurrently within a single thread. The heart of `asyncio` is its event loop, a mechanism that effectively orchestrates these tasks, switching between them without waiting for any one to finish before starting another. This efficient juggling act is made possible by coroutines, which allow developers to write code that pauses execution while awaiting an operation to complete, giving the event loop the freedom to tackle other tasks in the meantime.
While the concept of concurrent processing can seem complex, `asyncio` makes it fairly approachable. By using `asyncio.run`, you can kick off the event loop and define the core functions that handle your asynchronous operations. Functions like `await asyncio.sleep` allow you to model real-world delays (e.g., waiting for an external API response) without locking up the entire process.
This approach to handling translation requests can have a huge positive impact on performance. Instead of dealing with each request sequentially and potentially stalling while waiting for a response, `asyncio` allows the system to make better use of available resources and handle a larger workload. The result can be significantly faster response times, especially critical for services that aim to provide cheap and fast translation services, as well as in cases where quick turnaround times are needed for OCR operations. While implementing this kind of asynchronous pattern might require a shift in thinking from standard, sequential program design, the rewards in terms of efficiency and speed can be substantial. It's a notable tool for improving the scalability and responsiveness of AI-powered translation platforms.
Asynchronous processing using Python's `asyncio` library offers a compelling approach to handling numerous I/O-bound tasks simultaneously, like making API calls to retrieve translations from diverse AI services. This capability is especially valuable for building responsive translation applications that can gracefully manage heavy user loads.
One intriguing aspect of `asyncio` is its ability to significantly reduce latency in situations with many users. It achieves this by allowing one task to pause and relinquish control while waiting for an I/O operation to complete. This clever design leads to faster response times for translation requests, even during periods of high service usage.
The `asyncio` event loop manages tasks within a single thread, which simplifies concurrent programming compared to more traditional multi-threaded approaches. This inherent simplicity makes it easier to both implement and maintain translation services designed for high load and real-time responsiveness.
While `asyncio` offers a simpler path to concurrency, it leverages a cooperative multitasking model. This means that tasks explicitly cede control when appropriate. This can lead to a more predictable performance profile in translation systems where controlled execution is desirable, compared to the less predictable nature of thread scheduling.
Libraries that natively support `awaitable` objects, such as some HTTP clients often utilized for web scraping to gather OCR resources, work remarkably well with `asyncio`. This integration can result in more efficient and quicker data retrieval within translation workflows.
Coroutines, a core feature of `asyncio`, facilitate a high degree of concurrency without the traditional overhead of thread management. This is particularly advantageous when interfacing with external translation and OCR services, as these services frequently involve waiting for network responses.
Employing asynchronous processing techniques with `asyncio` can result in substantial cost reductions when scaling translation services. This arises from the ability to establish more concurrent connections to external APIs, consequently reducing the overall infrastructure required compared to synchronous solutions.
While powerful, testing and debugging `asyncio` applications can be more complex than traditional synchronous programs due to the added complexity of concurrent tasks. However, utilizing tools like `pytest-asyncio` can alleviate some of these challenges by offering a familiar testing framework for asynchronous operations.
Modern web frameworks like FastAPI, which offer support for asynchronous routes, can integrate seamlessly with `asyncio` for the creation of high-performance translation services. These frameworks enable handling many concurrent requests without blocking, enhancing the overall user experience.
Interestingly, while primarily focused on I/O-bound tasks, `asyncio` can be combined with other concurrency paradigms, like `multiprocessing`, to optimize CPU-bound operations, such as batching translation requests or handling computationally intensive OCR tasks. This hybrid approach opens up additional opportunities for performance enhancement within the AI translation pipeline.
Accelerating AI Translation 7 Python Optimization Techniques for High-Load Services - Optimizing Memory Usage through Cython Extensions
When dealing with demanding AI applications like high-volume translation services or OCR, optimizing memory usage becomes crucial for performance. This is where Cython can be a valuable asset. Cython allows us to write specific parts of our Python code in C, blending the strengths of both languages. This means faster code execution and, importantly, the potential to achieve much better memory management.
Cython's approach involves using `.pyx` files for the code meant to be optimized, alongside a `setup.py` file that handles the build process. Through this, developers can craft solutions that use the right data structures for each task and implement techniques like generators or utilizing class attribute slots for improved memory efficiency. This kind of fine-grained control over memory management becomes especially critical when dealing with a heavy load of translation requests.
The benefits are clear. By combining C's speed and Python's flexibility, Cython enables us to create more resource-efficient translation and OCR services. This is critical for maintaining smooth service delivery in high-load situations where response time is paramount. In short, Cython offers a potential path towards unlocking greater capabilities in AI translation, helping to ensure that these advanced technologies remain viable even under immense operational pressure.
Here's a rewrite of the provided text in a similar style and length, focusing on AI translation optimization through Cython:
When we consider accelerating AI translation services, particularly those that handle high volumes of requests or demand rapid response times (like fast translation or OCR), memory efficiency becomes paramount. Cython, a fascinating hybrid language, offers a compelling avenue to optimize memory usage within the Python ecosystem. Cython essentially allows us to bridge the gap between Python's ease of use and C's performance capabilities, essentially allowing developers to write specific components in C while preserving Python's high-level features elsewhere in the application.
The workflow typically involves writing optimization code in .pyx files, defining build configurations within a setup.py file, and then compiling. This hybrid approach can provide significant speedups (between 5 and 100x in some cases), making it especially beneficial for computationally intensive tasks within AI translation. For instance, one can imagine speeding up the translation process by efficiently manipulating arrays of text or accelerating OCR by using fast C-based image processing routines.
Cython allows for fine-grained control over memory through its ability to interact directly with C data types. By carefully managing data structures, developers can minimize memory overhead, a crucial consideration when handling the vast amounts of data found in NLP tasks. This degree of control over memory management, while potentially introducing some complexity, gives us significant leverage over performance.
The beauty of Cython lies in its seamless integration with NumPy. NumPy, a fundamental Python library in the world of AI and data science, handles large arrays, crucial for many AI translation tasks. By enabling seamless interactions with Numpy arrays, Cython can avoid the common memory pitfalls that can arise when handling complex data structures in a purely Python environment. It’s as if you get to keep the friendly face of NumPy while getting a huge boost in efficiency.
One might even consider integrating parallel computing into these Cython extensions using OpenMP. Parallelization can drastically reduce processing time for certain types of translation tasks and can be particularly important when considering translation from many languages at once or handling particularly large batches of text. It's interesting to think about the possibilities for splitting OCR operations across multiple cores.
Cython also allows us to leverage static type declarations. These declarations not only enhance runtime performance (less decision making at runtime) but also help guide the compiler towards more aggressive optimizations. In practice, this can mean faster translation speeds, especially when dealing with the repetitive nature of string operations and text formatting, which are abundant in these AI tasks.
It’s worth noting that Cython does allow for automatic memory management, though you also gain fine-grained control. It strikes a balance between the conveniences of automatic memory management that Python provides and the efficiency benefits of manual control. In other words, you don’t have to sacrifice the “Pythonic” aspects of development to get performance enhancements.
Of course, there are downsides. Debugging and understanding compiled extensions can be more challenging than pure Python code, potentially leading to a steeper learning curve. And there’s always the concern that the need for more developer intervention might be a roadblock to wider adoption. But when the gains are so substantial, these difficulties might be a worthwhile trade-off.
Cython’s ability to seamlessly interoperate with C libraries is another intriguing aspect. It's conceivable that this allows developers to directly utilize highly optimized algorithms written in C/C++, especially in the areas of OCR or advanced language modeling. This path would enable AI translation service developers to take advantage of existing work in these domains and combine it with the flexibility and ease of development Python provides.
In the rapidly evolving field of AI translation, optimizing for memory becomes crucial for both maintaining performance and controlling costs in high-load environments. Cython presents an appealing path towards reaching these objectives, offering a powerful tool in the quest for more efficient and faster AI translation services. While it may introduce additional complexity, the rewards in terms of enhanced performance and scalability can be significant, especially in scenarios like the development of specialized OCR tools.
Accelerating AI Translation 7 Python Optimization Techniques for High-Load Services - Utilizing Numba for Just-in-Time Compilation of NumPy Operations
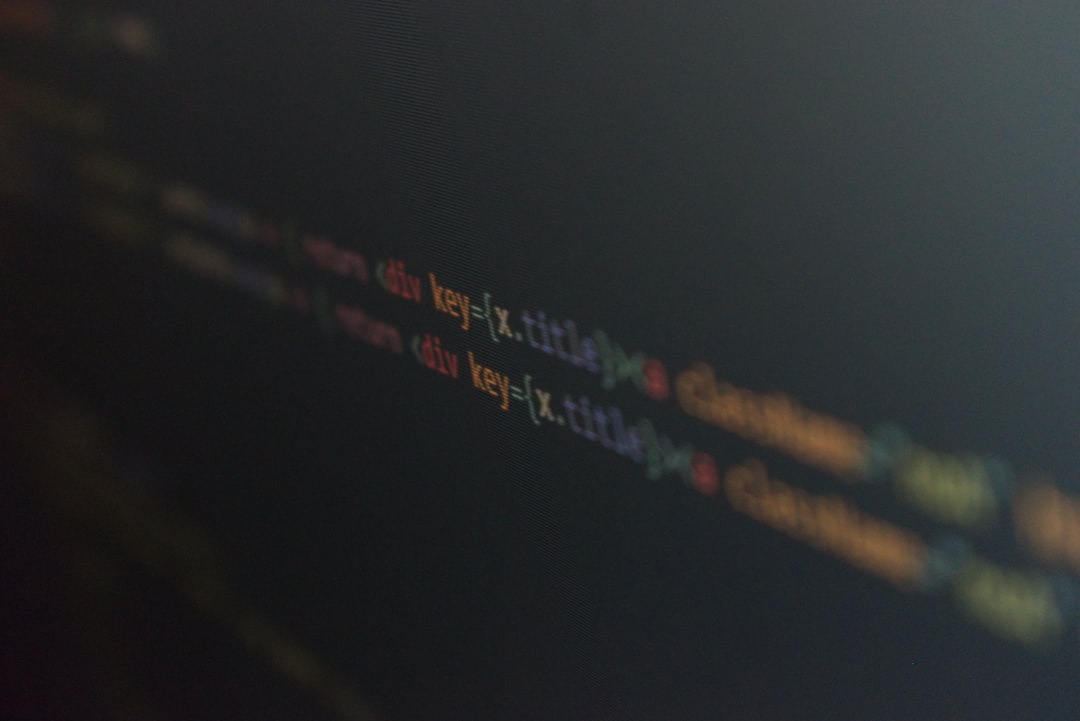
Leveraging Numba for just-in-time (JIT) compilation of NumPy operations can be a valuable approach to boosting the performance of AI translation services handling heavy workloads. Numba excels at speeding up numerical calculations by dynamically translating a portion of Python and NumPy code into highly optimized machine code during program execution. This technique is especially potent when dealing with mathematical operations and loops, aspects often central to tasks involving large arrays of data – common in AI translation and OCR. The speed benefits can be substantial, making it ideal for scenarios where swift processing is crucial. Implementing Numba often entails simply adding the `@njit` decorator to relevant functions, which keeps code changes to a minimum while still potentially delivering significant performance improvements. For developers aiming to enhance AI translation efficiency and handle a high volume of translation requests quickly, Numba offers a user-friendly yet potent path towards optimizing code, ultimately supporting the drive for fast and affordable translation services. While it does offer a valuable tool, it's important to acknowledge that the benefit of Numba is very much related to the specific code that is being optimized. It's not a magical panacea that automatically speeds up any arbitrary Python function.
Numba, an open-source Just-In-Time (JIT) compiler, can provide a 2 to 10-fold speed boost to NumPy operations. This is particularly useful in computationally demanding parts of AI translation, such as analyzing numerical data within a translation model. The JIT aspect is key here – Numba translates Python and NumPy code into optimized machine code on the fly, allowing it to accelerate array operations without needing to resort to the complexities of C or Cython. This streamlined optimization path is appealing for developers.
One surprising benefit of Numba is its automatic parallel code generation capability. This can come in handy when dealing with situations like translating multiple documents at once or processing batches of OCR operations, tasks where the ability to use multiple CPU cores in parallel can really speed things up. Numba also provides support for using GPUs to accelerate operations, like handling massive matrix operations found in translation models. This can provide substantial performance gains.
While offering many benefits, Numba only supports a subset of Python. Developers must consider this compatibility aspect when applying it to existing Python codebases, as certain libraries and data types might not be directly compatible. This can limit its immediate adoption in some scenarios. However, its impact on reducing memory overhead during array calculations is noteworthy. JIT compilation can optimize memory access patterns better than standard Python execution, a helpful attribute when working in high-load environments where memory can be a bottleneck.
Learning Numba is fairly straightforward compared to some other optimization approaches. Developers can gradually adapt existing functions to be Numba-compatible without huge rewrites, which can lower the barrier to entry for adopting this optimization approach. Benchmarking has shown that the performance benefits can be substantial, with some tasks seeing over a 90% reduction in execution time, especially in high-demand settings. This makes Numba an attractive optimization tool in this field.
Unlike some traditional JIT compilers, Numba accommodates dynamic typing and diverse workloads. This allows for adaptation when translation parameters change or as machine learning models evolve. This flexibility can be crucial. However, there's a caveat to consider: the extensive JIT compilation Numba performs can sometimes introduce overhead. For smaller datasets or simpler tasks, this overhead can potentially negate any performance gains. This is a factor to keep in mind when choosing to implement Numba. The best outcomes often involve matching Numba to tasks where its strengths—particularly around heavy NumPy computation and parallelization—can truly shine.
Accelerating AI Translation 7 Python Optimization Techniques for High-Load Services - Enhancing Performance with Multiprocessing Pools
Leveraging Python's multiprocessing capabilities through process pools can be a game-changer for AI translation services, particularly those under heavy load. By creating a pool of processes, we can distribute the workload of translating text or processing OCR data across multiple CPU cores. This concurrent processing strategy can significantly enhance performance, especially when dealing with a large volume of translation requests. The `Pool` class within the `multiprocessing` module provides helpful methods like `map` and `map_async`, making it straightforward to apply functions to large datasets concurrently.
However, this optimization path isn't without its drawbacks. One key consideration is process startup time, as launching a new process can be relatively slow. In certain situations, manually managing tasks and splitting the work can sometimes result in better overall performance than simply relying on the automatic parallelization offered by process pools. For example, if you have a fixed set of translations needing to be completed, you could divide these up into smaller groups and assign them to various cores more efficiently than the automatic distribution may perform.
Ultimately, for tasks heavily reliant on CPU resources, such as AI translation and OCR, adopting a multiprocessing strategy can lead to faster response times, a higher throughput of requests, and a more responsive user experience. It's a compelling technique for accelerating these crucial aspects of high-load AI services. Though it needs careful implementation to avoid introducing its own performance bottlenecks related to launching and managing the processes.
Python's `multiprocessing` module offers a way to boost performance by creating pools of processes. These pools distribute tasks across multiple cores, allowing for simultaneous execution—which can be really helpful for tasks like rapid AI translation, OCR, and situations where a lot of translations are needed quickly. You can control how many tasks each worker process handles, which can affect how much memory is used versus the time it takes to start new processes.
The `Pool` class gives you tools like `map` and `map_async`, which apply functions to lists of data concurrently. This can be great for processing batches of translation requests in parallel, though getting the process pools up and running can sometimes be slower than handling tasks one by one, especially if they are very small tasks.
If we have long-running processes, like ones that might be doing a lot of inferencing with a machine learning model, it makes sense to have each process load the model only once. Doing this can speed up the work being done in each process, and it aligns well with the idea of distributing the workload across multiple cores.
When you have a service that gets a lot of requests, and the processing is mostly limited by CPU speed, multi-processing is a strong contender. It leverages multiple cores to increase the overall speed of processing, enhancing the service's ability to handle a large volume of tasks simultaneously.
You might be happy to know that `Pool` is part of the standard Python library, meaning you don't need to install any extra packages. It's readily available and pretty simple to use. There are a few best practices that can help avoid pitfalls when using process pools, such as using context managers to ensure resources are released properly, using the `map` method for loops that can run concurrently, and being aware of how much memory each process is using.
The `multiprocessing` library is quite powerful, a key part of the Python ecosystem for unleashing parallel processing in applications. This can be extremely beneficial for tasks where time is a key constraint, such as transcription, where speed is essential but model changes aren't always possible or desired. We can simply apply the technique and speed up the process.
While it looks promising, it's not always a simple solution. There are a few things to keep in mind. For instance, while you can theoretically share data between processes through techniques like `multiprocessing.Array` or `Value`, this isn't always ideal. Each process has its own memory, and when tasks get complex, they sometimes need to serialize data to communicate. If not done carefully, this serialization step can become a bottleneck, potentially negating the benefits of parallelization. Plus, managing errors across multiple processes is a bit more involved than a single process. If one process crashes, it can affect the entire process if we aren't careful. And, the code can become a bit more complex to read and maintain because we have to manage communication and synchronization across processes. But the potential speed improvements can be quite large, and in many cases the drawbacks are manageable.
Essentially, process pools offer a pathway for maximizing the use of multiple cores and potentially speeding up translation services significantly. It can be a great tool to have in your toolbox when facing demanding, high-volume AI translation scenarios, particularly those that handle large volumes of requests or OCR, but it's best to weigh the complexity and benefits carefully.
Accelerating AI Translation 7 Python Optimization Techniques for High-Load Services - Streamlining Data Pipelines with Dask for Large-Scale Processing
Within the realm of AI translation, especially when dealing with the demands of high-volume services like fast translation or OCR, the need for efficient data processing becomes critical. Dask, a Python library specifically designed for large-scale data processing, offers a compelling solution to this challenge. By enabling parallel and distributed computation, Dask can tackle massive datasets with ease. This is especially important when the speed of translation or the efficiency of OCR is critical.
A key advantage of Dask is its ability to divide large datasets into smaller, manageable chunks. This approach facilitates processing datasets that might be too large to fit into a single computer's memory. This capability is crucial for services aiming to deliver near-instantaneous translations or OCR results, even when handling substantial data volumes.
Furthermore, Dask blends seamlessly with familiar Python data tools like NumPy and pandas. This compatibility minimizes the disruption to existing codebases, allowing developers to integrate Dask into their AI translation workflows without requiring major rewrites. This smooth transition and the ability to seamlessly scale the number of workers as needed make Dask a more accessible solution for those seeking improvements.
Dask also offers a user-friendly interface for monitoring and managing tasks. This allows users to observe the status of their data processing pipelines in real-time. This visibility provides developers with a better understanding of how their translation systems are performing.
However, it's crucial to acknowledge that using Dask, especially for large-scale systems, introduces complexities that need careful consideration. Implementing and managing distributed systems can be challenging. While the rewards for performance and scalability can be substantial, developers need to be aware of the associated challenges before adopting Dask in a production setting.
Dask, a Python library built for tackling large-scale data processing, has shown promising results in the realm of AI translation. It operates by breaking down massive datasets into smaller, manageable chunks, and then efficiently distributing the processing across multiple machines or cores. This approach is particularly relevant when dealing with the vast amounts of textual data involved in AI translation, potentially leading to faster translation speeds and reduced costs for service providers.
One of Dask's key features is its ability to scale effortlessly, handling everything from single-machine tasks to complex clusters with thousands of nodes. This adaptability is important for services handling high volumes of requests, like those seen in cheap translation platforms or OCR tools. You can scale up or down based on the translation workload without major code changes. Further, Dask uses "lazy evaluation" meaning it builds a computational plan before actually running it. This intelligent strategy helps to minimize unnecessary computations, a valuable optimization in the often intricate world of translation pipelines. Imagine optimizing how a translation model is applied to the text.
Dask's inherent parallel processing capabilities are also extremely valuable in this context. Tasks that might typically require hours to complete using traditional methods can potentially be condensed into mere minutes with Dask. This kind of speedup is especially useful when handling multiple translation requests simultaneously or performing OCR on lengthy documents, as seen in tools like automated document translators. Dask's dynamic task scheduler also smartly adapts to changes in available computational resources, making sure that translation requests are addressed efficiently, even under intense loads. This kind of dynamic adaptability can be really helpful in preventing service disruptions during periods of high demand for translation services.
Dask plays nicely with familiar Python data science libraries like NumPy and Pandas. This means developers can apply their existing knowledge and work styles when building translation systems using Dask. If a developer is used to working with Pandas dataframes, they can use Dask Dataframes with minimal code changes to get performance improvements. This compatibility extends beyond mere data processing, with Dask offering support for scalable machine learning using libraries like Dask-ML. This allows the translation models themselves to benefit from Dask’s speed-enhancing features, contributing to faster translation output and training times.
Another interesting aspect is how Dask handles memory limitations. When memory becomes tight, it cleverly moves data to disk to ensure the computation doesn’t crash. This is particularly important for AI translation tasks that deal with very large datasets or complex models. The potential to keep translations going without crashing is a notable win. Dask can even do real-time data processing, making it attractive for live translation services where speed is a crucial factor in customer experience. Its flexibility extends to managing the full translation pipeline, including data intake, cleaning, processing, and the model inference itself, enabling more holistic performance improvements.
While adopting a new library like Dask might take some effort, it presents an attractive path towards creating more efficient and cost-effective AI translation solutions. It is possible to shift from more expensive or resource-intensive solutions to Dask's distributed computing for cost savings. This could enable services to offer cheaper translations and compete more effectively in a dynamic market. While the transition to Dask might not be immediate or universally appropriate for every translation project, its potential benefits, particularly in demanding environments, make it worth considering as a powerful tool for optimizing AI translation.
Accelerating AI Translation 7 Python Optimization Techniques for High-Load Services - Employing TensorFlow Lite for Efficient Model Deployment
TensorFlow Lite, now called LiteRT, provides a promising approach for deploying machine learning models, especially in scenarios like AI translation and OCR, where edge devices are common. The core focus of LiteRT is optimization for resource-constrained environments, tackling issues like latency and limited memory, which are paramount when dealing with high service loads. LiteRT's integration with libraries like XNNPACK improves inference performance, particularly on CPUs, potentially delivering 2 to 3 times faster floating-point calculations during neural network inference. The availability of pretrained models within LiteRT also simplifies development, allowing developers to add machine learning features to mobile or embedded applications without the overhead of training new models from scratch. This focus on efficiency, speed, and ease-of-use makes it a strong candidate for services needing to quickly and cheaply deliver AI-driven translation and OCR capabilities. While the improvements are welcome, it's important to remember that any optimization technique needs to be carefully matched to the specific task. However, LiteRT’s ability to bring powerful AI models to resource-limited environments is certainly a significant development.
TensorFlow Lite, now also known as LiteRT, is actively being developed to refine model runtime and optimization tools, particularly useful when considering how to deploy AI models, like those for translation, on devices with limited resources. Edge devices, often found in mobile phones or embedded systems, are typically constrained by memory and processing power. This constraint drives the need for techniques that allow for machine learning models to operate within these limitations without sacrificing, or at least minimizing, accuracy.
LiteRT, alongside the TensorFlow Model Optimization Toolkit, provides essential tools for achieving this goal. Tools for model optimization are critical since they allow us to deploy models in environments where we are restricted by factors like available power, memory, and processing speed. The XNNPACK library, for instance, is integrated into TensorFlow Lite to improve inference performance on CPUs. In some cases, this integration leads to 2-3 times faster inference for floating-point operations, which can be really helpful when aiming for faster translation speeds.
LiteRT includes pre-trained models that are ready to be used. This can be a huge time-saver for developers who want to add machine learning features to applications without having to train or build a new model from scratch. It can be a very effective way to quickly build applications that leverage AI translation or OCR capabilities.
The Model Optimization Toolkit contains features focused on lowering latency and inference cost, which is vital for efficiently deploying translation models in a variety of settings, including cloud and edge devices. The idea is to reduce the overall complexity of a model, a goal that also impacts areas like latency, power consumption, and memory usage, factors that are particularly important when deploying models onto edge devices.
Following the optimization of a TensorFlow Lite model, the accuracy can be evaluated with a specific function using a collection of test images, confirming the effectiveness of the adjustments and confirming that the speed improvements haven't resulted in a degradation of the quality of the model's output.
One of the neat things about TensorFlow Lite is that it facilitates the usage of specialized hardware for faster inference. This is important since performance can vary greatly depending on the hardware that is being used, a factor that needs to be considered when trying to ensure that an AI translation application performs well across different devices.
Model optimization strategies often involve considering things like processing limitations, memory constraints, the amount of power used, network usage limitations, and the storage space of a target device. These limitations play a crucial role when deciding on a particular optimization approach. While it might seem like a lot to consider, it ultimately ensures that models are as efficient as possible in the real-world, a crucial factor for keeping services like AI translation viable on a large scale.
In conclusion, LiteRT provides a solid foundation for deploying optimized AI translation models on diverse devices, potentially contributing to a broader adoption of these technologies by facilitating their accessibility across a wider range of hardware platforms and minimizing their performance limitations, leading to a future where quick and accurate translation is a reality.
More Posts from aitranslations.io: